You can easily insert images into Excel spreadsheets and edit them with macro code. Here's how to insert a picture into a cell using VBA in Excel .
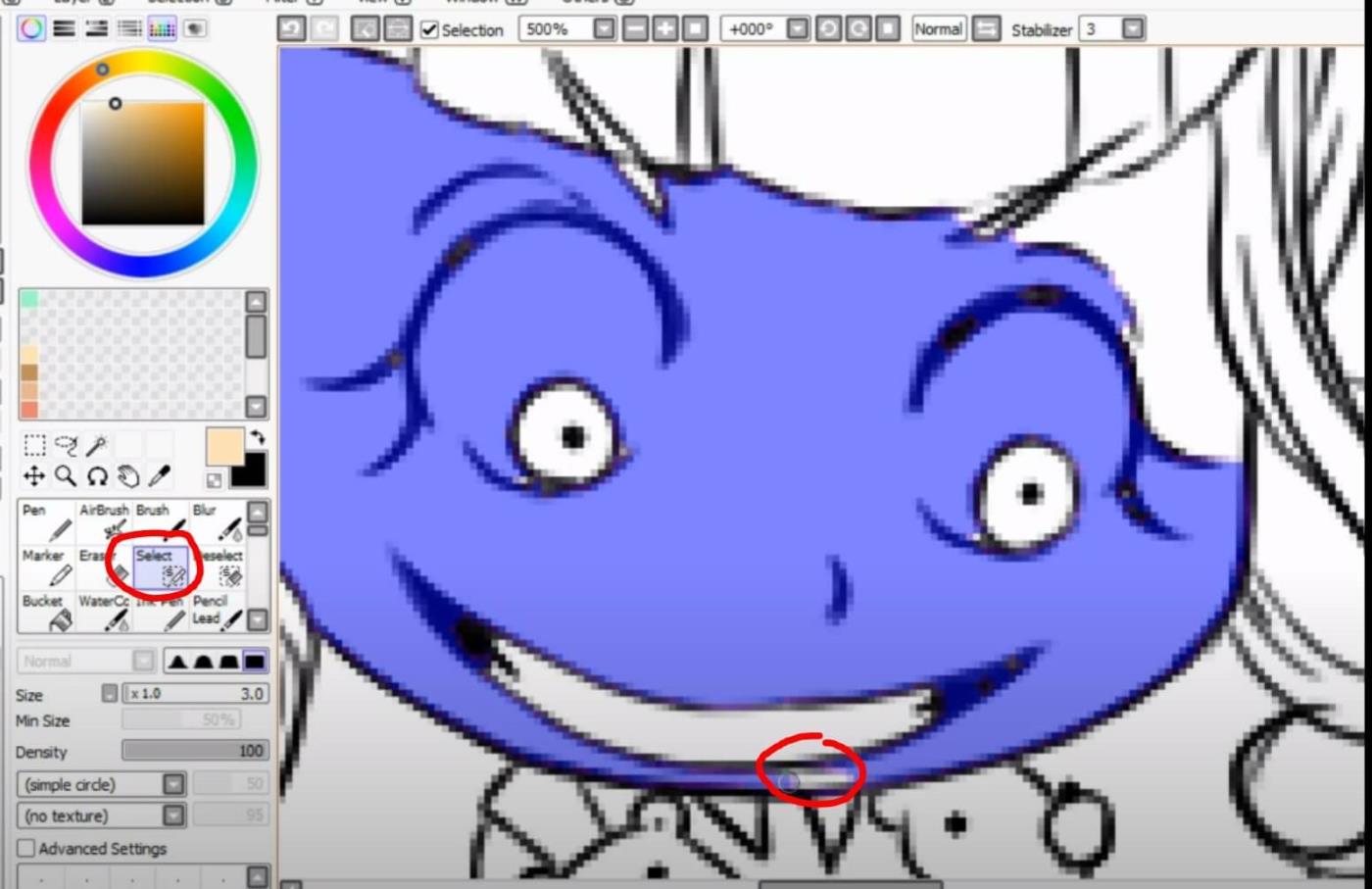
Visual Basic for Applications, abbreviated as VBA, is a form of Visual Basic 6 integrated into Microsoft Office programs. Through coding, VBA allows you to automate tasks in Office applications, including Excel. In some cases, you can even add new features to Excel using VBA.
Even though you need to work with code to use VBA, that doesn't mean VBA is full of letters and numbers. With VBA in Excel, you can create a macro that allows you to insert a picture into a cell or range of cells. Here's what you need to know about inserting pictures into cells in Excel using VBA.
How to insert pictures into cells using VBA in Excel
To create a macro that inserts a picture into an Excel cell using VBA, you really don't need any advanced Visual Basic knowledge. All you need to do is turn on Developer tools, create a macro, and paste the appropriate code. Of course, you can also insert images into Excel without using VBA. However, in this article, we will focus on VBA.
1. Turn on Developer tools
To use VBA in Excel, you need to enable Developer tools. This action will enable the Developer tab in the ribbon, which is disabled by default.
- Open Excel .
- Go to the File menu .
- Click Options at the bottom of the screen. The Excel Options window will appear.
- In Excel Options, go to the Customize Ribbon tab .
- In Main Tabs , check Developer .
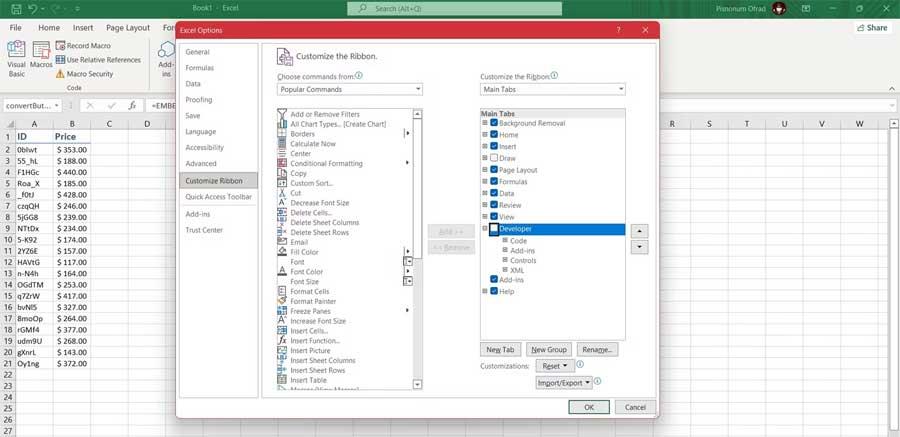
Now Developer tools, including VBA access, are enabled. You don't need to do this every time you want to use VBA in Excel. Developer tools will always be enabled until you disable them.
2. Create macros and insert code
- In Excel, go to the Developer tab .
- In the Code section, select Macros .
- In the new window, enter your macro name in Macro name . The article will use insertPhotoMacro .
- Click Create .
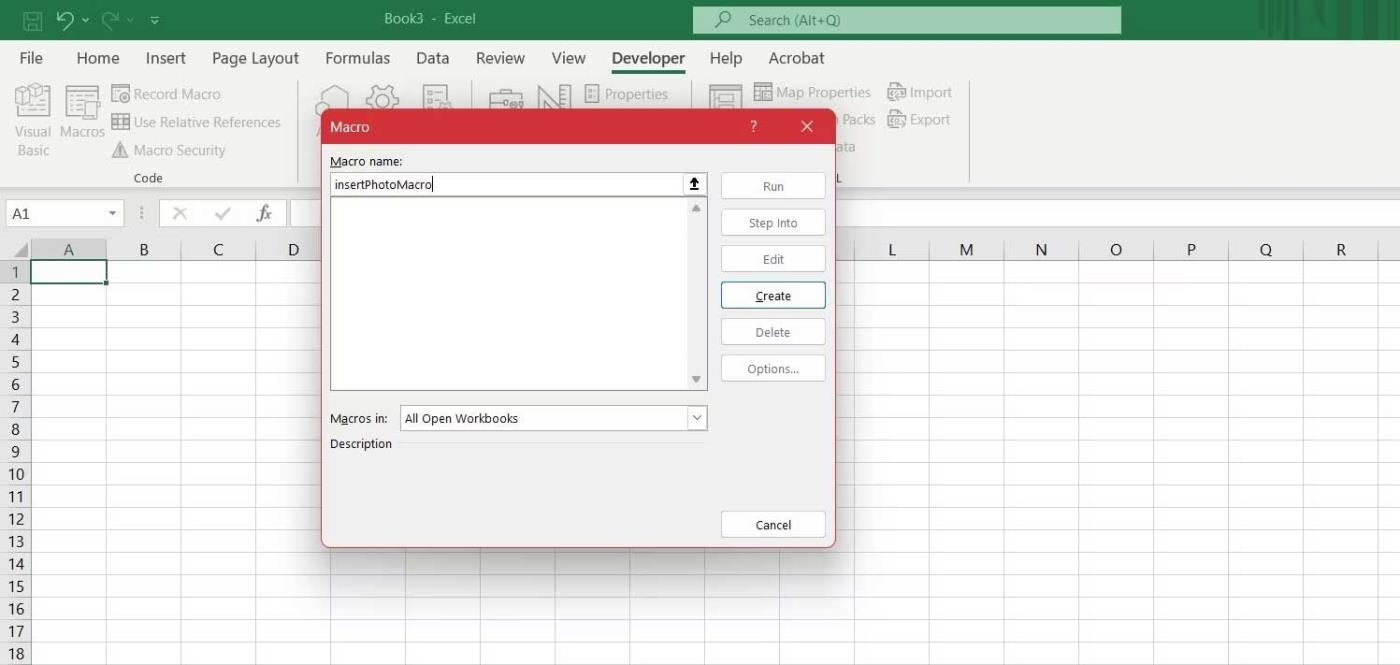
After clicking Create, the VBA window will open and display the code for your macro. Currently, the code will consist of 2 lines: a Sub to start the macro and an End Sub to end it.
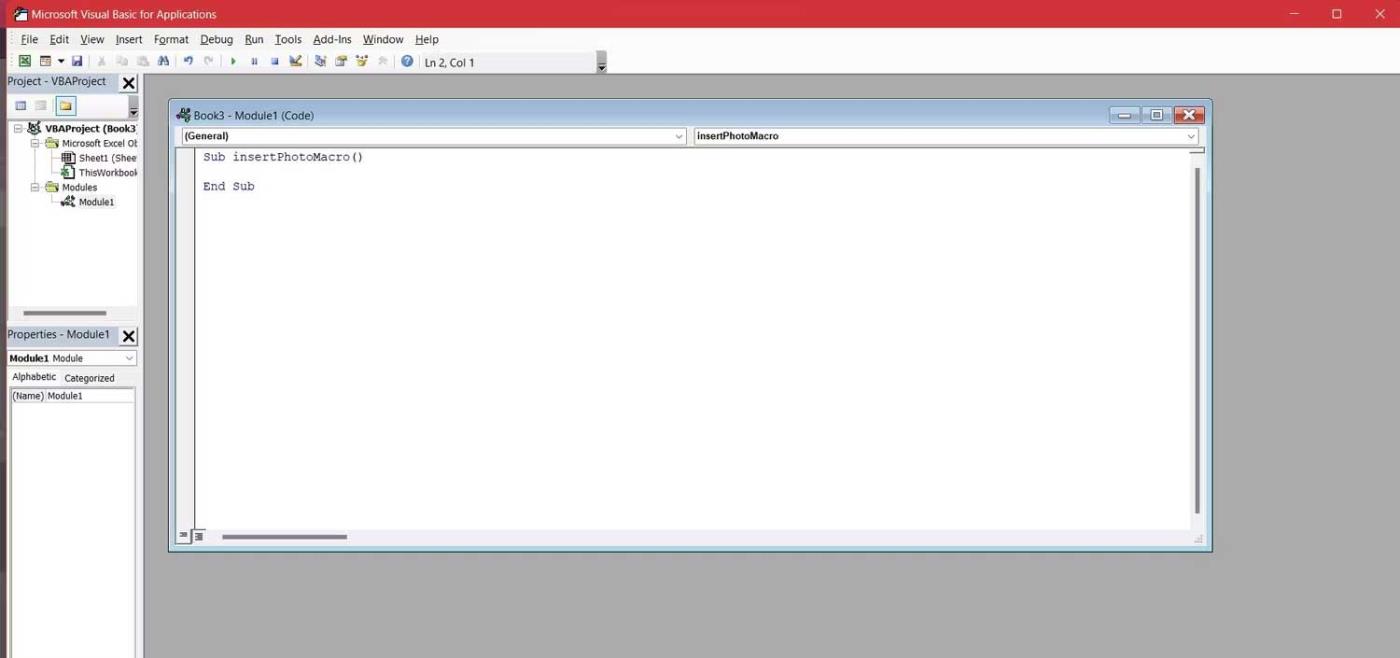
Add a little code to this macro. Add the following line of code between the two lines:
Dim photoNameAndPath As Variant
Dim photo As Picture
photoNameAndPath = Application.GetOpenFilename(Title:="Select Photo to Insert")
If photoNameAndPath = False Then Exit Sub
Set photo = ActiveSheet.Pictures.Insert(photoNameAndPath)
With photo
.Left = ActiveSheet.Range("A1").Left
.Top = ActiveSheet.Range("A1").Top
.Width = ActiveSheet.Range("A1").Width
.Height = ActiveSheet.Range("A1").Height
.Placement = 1
End With
The final code will look like this:
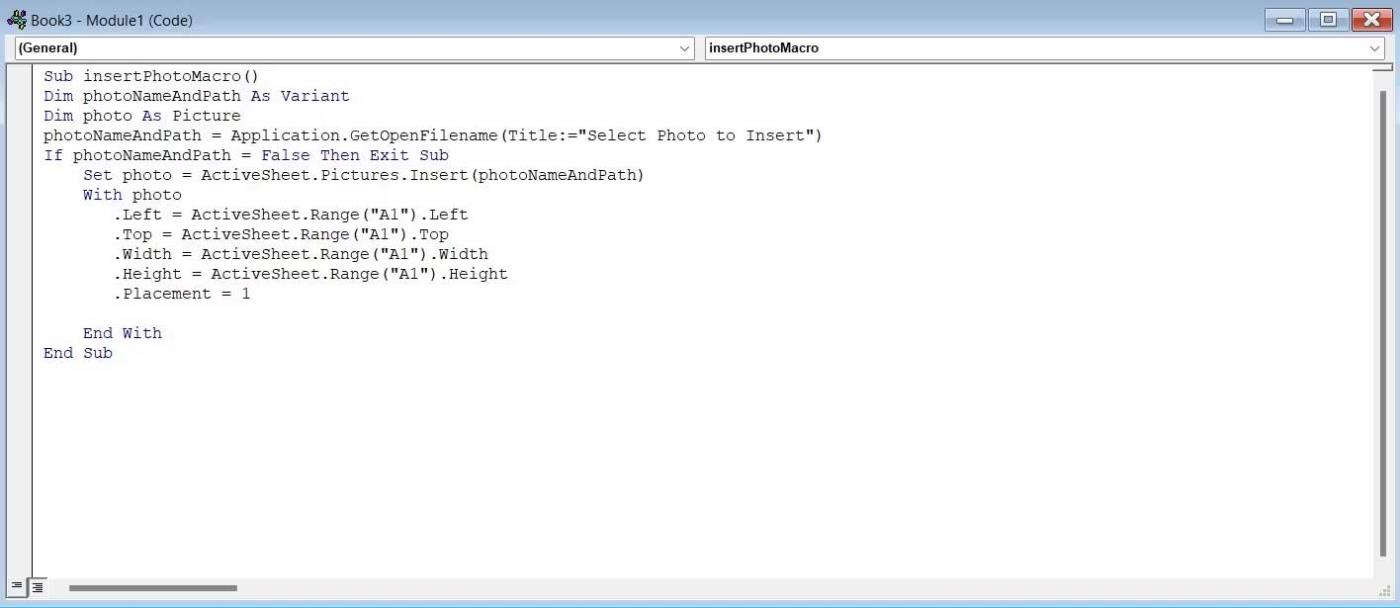
You don't need to worry about saving this process. Any changes you make in VBA are saved immediately.
Now it's time to see the code in action:
- Close the VBA window.
- Go to the Developer tab in Excel.
- Select Macros from the Code section.
- Highlight the macro you just created.
- Click Run .
Now a message will open asking you to locate the image file you want to insert. Select the photo, then click Open. You will now see the image in cell A1.
Note, Excel will shrink the image so it fits in cell A1. You can change it and change the code to insert images into other cells, even a range of cells. In the next section, we will separate the code and explain the parameters.
3. Split code
For VBA code to work the way you want, you need to understand it. When doing this, you can change the code to insert images into any cell at any size.
Sub insertPhotoMacro()
Dim photoNameAndPath As Variant
Dim photo As Picture
photoNameAndPath = Application.GetOpenFilename(Title:="Select Photo to Insert")
If photoNameAndPath = False Then Exit Sub
Set photo = ActiveSheet.Pictures.Insert(photoNameAndPath)
With photo
.Left = ActiveSheet.Range("A1").Left
.Top = ActiveSheet.Range("A1").Top
.Width = ActiveSheet.Range("A1").Width
.Height = ActiveSheet.Range("A1").Height
.Placement = 1
End With
End Sub
When code begins, the Dim statement is used to determine the variable type. We have two variable types: photoNameAndPath and photo . First the variable and then the image.
From there, the photoNameAndPath variable runs and it will open an application to get the location of the photo file. This is done via Application.GetOpenFileName . The Title parameter is optional. The content inside it is displayed as the window name.
Using If photoNameAndPath = False Then Exit Sub , if an invalid or empty address is given, the process will end. However, if a suitable file is provided, Set photo = ActiveSheet.Pictures.Insert(photoNameAndPath) indicates that the picture should be set as a variable as originally defined. It will be inserted into the active worksheet.
Finally, use With photo and the 5 lines after it to determine the location of the photo. .Left and .Top refer to the starting position, while .Width and .Height refer to the ending position. If you intend to insert images into another cell or a range, these are the lines you should change.
.Placement indicates that the picture should be resized to fit the cell or inserted freeform. Setting it to 1 will resize it according to the cell.
Finally, the above code uses End With , then End Sub to close the macro. Now you can change the variables photoNameAndPath and photo to any other name you like. Just remember to keep the names consistent throughout the code.
Above is how to use VBA to insert images into cells in Excel . Hope the article is useful to you.